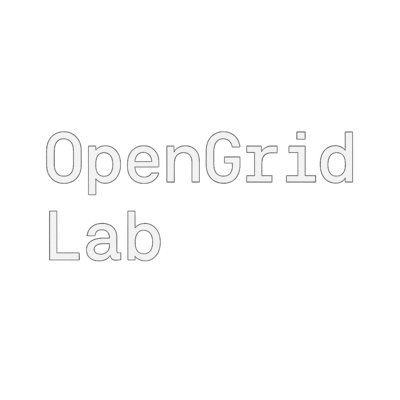
How to Vibe Code a Working SSH Script
Sometimes you don’t want to architect a reusable CLI toolkit.
You just want to vibe into a working shell script that gets the job done.
SSH scripts are a great candidate. They can: - Connect to remote boxes fast - Run repeatable commands - Automate uploads or deploys - Save you from retyping 5-minute aliases
Here’s how to casually code one without making it more complex than it needs to be.
Step 1: Pick Your Targets
Assume you have a couple of remote servers:
web1.mydomain.com
db1.internal.lan
You could write this all inline, but even lazy scripts deserve variables.
#!/bin/bash
WEB_HOST="web1.mydomain.com"
DB_HOST="db1.internal.lan"
Step 2: Make SSH Talk
Here’s a simple one-liner to connect:
ssh user@$WEB_HOST
But let’s make that a function so we can call it whenever:
connect_web() {
ssh user@$WEB_HOST
}
Step 3: Automate Uploads (Just Enough)
Want to copy a file to your remote box? You don’t need Ansible. You need scp
.
deploy_web() {
scp ./site.zip user@$WEB_HOST:/var/www/html/
ssh user@$WEB_HOST "cd /var/www/html && unzip -o site.zip"
}
That’s a real-world deploy script in 6 lines.
Step 4: Add Some Flags (Optional)
You can even make it take arguments if you want to switch targets or modes:
case "$1" in
web) connect_web ;;
deploy) deploy_web ;;
*) echo "Usage: $0 [web|deploy]" ;;
esac
Step 5: Make It Runnable
Save your script as remote.sh
, then run:
chmod +x remote.sh
./remote.sh web
Congrats. You now have: - A working SSH shortcut - A lazy deploy system - No YAML in sight
Why This Works
You didn’t overthink it.
You didn’t reach for a framework.
You wrote code that works with muscle memory.
That’s vibe coding.
Last updated: 2025-04-09 UTC